How to Claim Your Free $25 Monthly xAI API Credits for Grok2: A Complete Guide

xAI has announced an exciting offer providing $25 monthly API credits for their Grok2 beta service until the end of 2024. This comprehensive guide walks users through the entire process, from initial account creation to implementing practical API calls. We cover both shell script and Python implementations, making it accessible for users of all technical levels. The tutorial includes detailed steps for account setup, API key generation, and code examples for both methods, complete with security best practices and troubleshooting tips. Whether you’re a developer, researcher, or AI enthusiast, this guide helps you to quickly start experimenting with Grok2-beta while making the most of your monthly credits through the x.ai console platform.
xAI’s highly anticipated Grok2 has entered its beta phase with an enticing proposition for developers and AI enthusiasts. The company is rolling out $25 worth of monthly API credits, making the service accessible until the end of 2024.
We’ll show you how to profit from this offer and try out Grok2 yourself. Here is how this works:
First you’ll need to create an account with xAI, claim your free credits, and create an API key.
Then you can start experimenting with the API through shell scripts or by using python. We’ll show you exactly how this is done from account creation all the way to python scripts. Interested? Then read on…
Getting Started with xAI Console
Our journey begins at https://console.x.ai, where you’ll be presented with a login screen. For first-time users, account creation is necessary, while existing users can simply sign in. The platform offers multiple authentication methods: email registration, X (formerly Twitter) authentication, or Google sign-in. This guide focuses on the email registration process, which provides the most straightforward approach for new users.
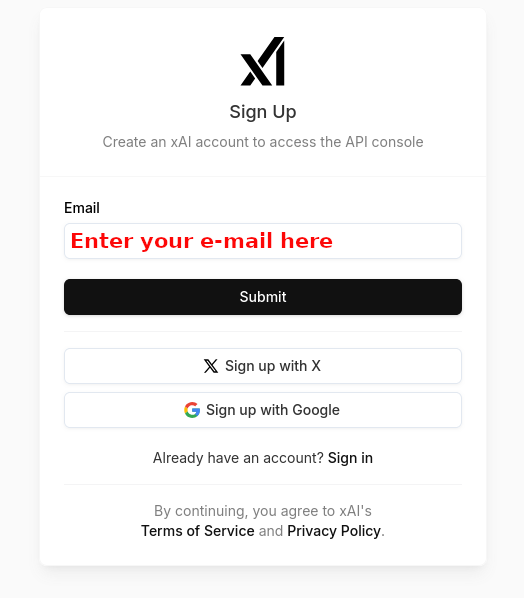
Account Creation and Verification
During the email registration process, you must provide a valid email address as xAI will send a verification link. After submitting your email, check your inbox for the verification link from xAI. Click the link to proceed with the account setup. You’ll be prompted to fill in your account details, though you have the flexibility to skip non-essential information if desired.

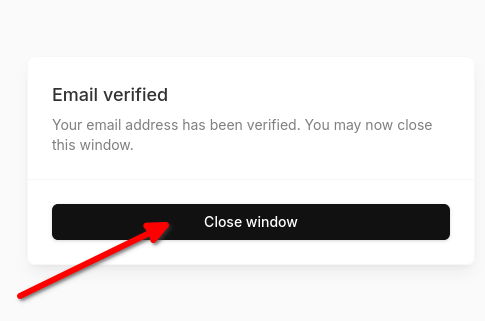
Clicking the link opens a new page where your account is verified. Once this is done, you can close the newly opened page. When you return to the sign-up page, the process will have clicked continue already. If not, you now can do that manually.
Terms of Service Agreement
Before proceeding, you must explicitly agree to two important conditions:
- The Enterprise Terms of Service and Privacy Policy
- Age verification confirming you’re over 18 years old
Both checkboxes must be selected to continue – this is a mandatory step that ensures compliance with legal requirements and service conditions.
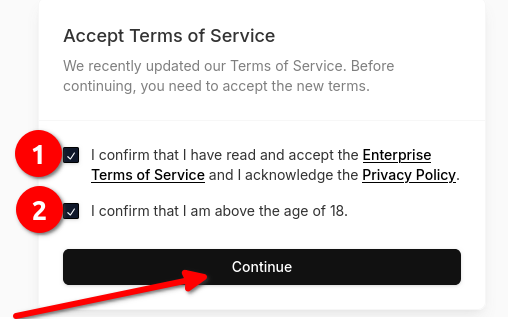
API Key Generation
Upon entering your account dashboard, you’ll notice a prominent banner with a “Generate API Key” button.

Clicking this button will take you to a screen titled “You are ready to build,” where your unique API key will be displayed. This is a critical moment in the setup process – the API key shown must be copied and stored securely, as it won’t be displayed again in your account interface.

Accessing Your Credits
Upon reaching your account’s home screen, you’ll notice a distinctive blue circle displaying your $25 free credits. These credits automatically renew at the beginning of each month until the end of December 2024, providing consistent access to the Grok2 beta API throughout the promotional period.
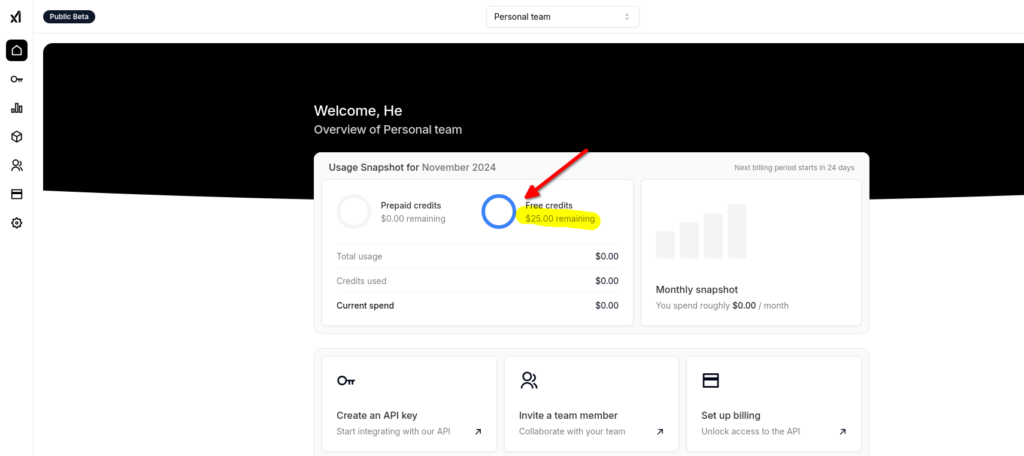
Testing Grok2-Beta: Two Approaches
There are two main methods to test your Grok2-beta access: using a shell script or implementing a Python solution. Let’s explore both options in detail.
You can either copy the scripts directly from this website or you can download them from GitHub: https://github.com/TheAIObs/groktest/
Method 1: Shell Script Implementation
For users comfortable with command-line interfaces, the shell script method is straightforward. This approach works on:
- Linux systems
- macOS
- Windows (using Windows Subsystem for Linux)
Prerequisites:
- Verify curl is installed (typically pre-installed on modern systems)
- Access to a terminal or command prompt
Steps:
- Create a new file named ‘testgrok.sh’:
#!/bin/bash
curl https://api.x.ai/v1/chat/completions -H "Content-Type: application/json" -H "Authorization: Bearer PUT_API_KEY_HERE" -d '{
"messages": [
{
"role": "system",
"content": "You are a test assistant."
},
{
"role": "user",
"content": "Testing. Just say hi and hello world and who you are and nothing else."
}
],
"model": "grok-beta",
"stream": false,
"temperature": 0
}'
2. Replace “PUT_API_KEY_HERE” with your actual API key in the script. The API key should have the form xai-[a lot of random-characters]
3. Save the file and make the script executable:
> chmod +x testgrok.sh
Instead of copying the script, you can also download it from GitHub: https://github.com/TheAIObs/groktest/
Method 2: Python Implementation
The Python approach offers more flexibility and is platform-independent. Here’s how to get started:
Setting Up Python:
- Windows: Download from python.org and run the installer
- macOS: Use Homebrew (‘brew install python3’) or download from python.org
- Linux: Usually pre-installed, or use package manager (‘apt install python3’)
Also make sure that you have installed the OpenAI library for python. You can do that with
> pip install openai
There are two ways to handle the API key with the Python script:
1. Direct Implementation:
- Insert the API key directly in the script
- Less secure but more convenient for personal use
Create a file testgrok_direct.py with the following content:
import os
from openai import OpenAI
XAI_API_KEY = "PUT_API_KEY_HERE"
client = OpenAI(
api_key=XAI_API_KEY,
base_url="https://api.x.ai/v1",
)
completion = client.chat.completions.create(
model="grok-beta",
messages=[
{"role": "system", "content": "You are Grok, a chatbot inspired by the Hitchhikers Guide to the Galaxy."},
{"role": "user", "content": "What is the meaning of life, the universe, and everything?"},
],
)
print(completion.choices[0].message)
Save the file and run it with
> python3 testgrok_direct.py
2. Environment Variable:
Create a file testgrok_env.py with the following content:
import os
from openai import OpenAI
XAI_API_KEY = os.getenv("XAI_API_KEY")
print(XAI_API_KEY)
client = OpenAI(
api_key=XAI_API_KEY,
base_url="https://api.x.ai/v1",
)
completion = client.chat.completions.create(
model="grok-beta",
messages=[
{"role": "system", "content": "You are Grok, a chatbot inspired by the Hitchhikers Guide to the Galaxy."},
{"role": "user", "content": "What is the meaning of life, the universe, and everything?"},
],
)
Save the file and run it with
> export XAI_API_KEY='YOUR_KEY_HERE'
> python3 testgrok_env.py
Instead of copying the scripts, you can also download them from GitHub: https://github.com/TheAIObs/groktest/
Enhanced Python Implementation
For a more user-friendly experience, we’ve created an enhanced version that accepts command-line arguments:
Create a file chatgrok.py with the following content:
import os
import argparse
from openai import OpenAI
def parse_arguments():
parser = argparse.ArgumentParser(description='Interact with Grok-beta API')
parser.add_argument('question', type=str, help='The question to ask Grok')
parser.add_argument('-s', '--system', type=str,
default="You are Grok, a chatbot inspired by the Hitchhikers Guide to the Galaxy.",
help='Custom system prompt (optional)')
return parser.parse_args()
def setup_client():
XAI_API_KEY = os.getenv("XAI_API_KEY")
if not XAI_API_KEY:
raise ValueError("XAI_API_KEY environment variable not set")
return OpenAI(
api_key=XAI_API_KEY,
base_url="https://api.x.ai/v1",
)
def get_grok_response(client, system_prompt, question):
try:
completion = client.chat.completions.create(
model="grok-beta",
messages=[
{"role": "system", "content": system_prompt},
{"role": "user", "content": question},
],
)
return completion.choices[0].message.content
except Exception as e:
return f"Error: {str(e)}"
def main():
args = parse_arguments()
client = setup_client()
print(f"\nSystem Prompt: {args.system}")
print(f"Question: {args.question}")
print("\nGrok's Response:")
response = get_grok_response(client, args.system, args.question)
print(response)
if __name__ == "__main__":
main()
This implementation allows for quick, one-line queries without modifying the script for each new question.
This script is a bit more complicated, but it includes:
- Argument parsing for both question and optional system prompt
- Better error handling
- Structured functions for better maintainability
- Clear output formatting
- Environment variable checking
- Exception handling for API calls
In order to try it, you can either copy the script from here or download it from GitHub: https://github.com/TheAIObs/groktest/
This script can be used in two ways:
- With default system prompt:
> python3 chatgrok.py "What is the meaning of 42?"
and grok2 will answer something like that:
System Prompt: You are Grok, a chatbot inspired by the Hitchhikers Guide to the Galaxy.
Question: What is the meaning of 42?
Grok's Response:
Ah, the age-old question about the meaning of 42. According to the Hitchhiker's Guide to the Galaxy, 42 is the "Answer to the Ultimate Question of Life, The Universe, and Everything." However, the actual question itself remains unknown. It's a humorous way of saying that the answer to the big questions in life is often elusive or nonsensical.
In essence, the number 42 has become a symbol of the quest for understanding the mysteries of existence. It reminds us that sometimes the journey to find meaning is more important than the answer itself, and it's perfectly fine that some questions might not have straightforward answers. Remember, as Douglas Adams put it, "Don't Panic!" and enjoy the adventure of figuring things out along the way!
Or you can specify a system prompt as well:
> python3 chatgrok.py -s "You are a helpful math tutor" "What is calculus?"
After which grok2 will give you an answer similar to the following one:
System Prompt: You are a helpful math tutor
Question: What is calculus?
Grok's Response:
Calculus is a branch of mathematics that deals with rates of change and how things change over time or over a continuous space. Here's a brief overview of its key components:
1. **Limits**: Calculus starts with the concept of limits, which helps in understanding the behavior of functions as they approach specific points or infinity. This concept underpins the entire field.
2. **Differential Calculus**:
- **Derivatives**: This part of calculus focuses on derivatives, which measure how a function changes as its input changes. Essentially, it calculates the slope of the tangent line to a curve at any given point. Derivatives are crucial for:
- Finding rates of change (e.g., velocity from position in physics).
- Optimization problems (maxima and minima).
- Linear approximations.
3. **Integral Calculus**:
- **Integrals**: This deals with accumulation or the area under curves. Integrals can be used to:
- Compute areas, volumes, and other quantities that accumulate over an interval.
- Solve differential equations by finding the antiderivative.
- **Definite Integrals**: Represent the net accumulation of quantities over an interval, often visualized as the area between the curve and the x-axis.
- **Indefinite Integrals**: Give the antiderivative of a function, which is a function whose derivative is the original function.
4. **Fundamental Theorem of Calculus**: This theorem links differentiation and integration, stating that differentiation and integration are inverse processes:
- It provides a way to compute definite integrals using antiderivatives, making calculations much simpler.
5. **Applications**: Calculus has widespread applications:
- **Physics**: Motion, electricity, magnetism, fluid dynamics.
- **Economics**: Optimization, consumer and producer surplus, elasticity.
- **Engineering**: Designing systems, control theory, signal processing.
- **Medicine**: Modeling biological systems, pharmacokinetics.
6. **Advanced Topics**: Beyond basic calculus, there are multivariable calculus, which deals with functions of more than one variable, vector calculus, differential equations, and more specialized areas like complex analysis.
Calculus provides the language and tools to describe, analyze, and understand change in a precise manner, which is why it's fundamental in many scientific, engineering, and economic models. It's often seen as challenging due to its abstract nature and the need to grasp concepts like infinitesimals or infinitely small changes, but once understood, it becomes an incredibly powerful tool.
Security Considerations
When working with API keys:
- Never commit API keys to version control: API keys should never be included in your Git repositories or any public code sharing platforms. Instead, use configuration files that are explicitly listed in your .gitignore file.
- Use environment variables when possible: Environment variables provide a secure way to store sensitive information outside your code. They can be easily set and modified without changing your application code, and they work across different deployment environments.
- Keep backup copies of your key in a secure location: Store your API key in a password manager or secure note-taking system. Consider using tools like LastPass or 1Password for team environments where multiple developers need access.
- Regularly monitor your API usage through the console: Set up regular checks of your API usage patterns through the x.ai console. This helps detect any unauthorized usage and ensures you’re staying within your credit limits.
- Consider using a .env file for local development: Create a separate .env file for storing environment variables during local development. Remember to add .env to your .gitignore file and provide a template file (e.g., .env.example) with dummy values for team collaboration.
Best Practices for Testing
- Start with simple queries to verify functionality: Begin with basic “Hello, World” style queries to ensure your setup is working correctly. This helps isolate any potential issues before moving to more complex implementations.
- Monitor credit usage during testing: Keep track of how many credits each type of query consumes. Set up a spreadsheet or logging system to document credit usage patterns and help plan for future scaling.
- Keep track of successful and failed requests: Implement proper logging mechanisms to record all API interactions. This includes request parameters, response times, and any error messages, which will be invaluable for debugging and optimization.
- Document any error messages or unexpected behavior: Create a detailed error log with timestamps and full context of each issue encountered. This documentation will help identify patterns and assist when seeking support from the xAI team.
- Test different query types to understand the API’s capabilities: Experiment with various query formats, lengths, and complexity levels. This helps understand the API’s strengths and limitations, allowing you to optimize your implementation accordingly.
Troubleshooting Common Issues
- Verify API key is correctly formatted: The API key should follow xAI’s specific format requirements. Check for any accidental whitespace or special characters that might have been introduced during copying.
- Check script permissions (for shell script): Ensure your shell scripts have the correct executable permissions (chmod +x). On Unix-based systems, verify the file ownership and group permissions match your user account.
- Ensure Python environment is properly configured: Confirm you’re using the correct Python version and all required dependencies are installed. Consider using virtual environments to avoid conflicts with other Python projects.
- Confirm curl is installed and functioning: Test curl with a simple HTTP request to verify it’s working correctly. On Windows, ensure the PATH environment variable includes the curl executable location.
- Monitor network connectivity: Check your network stability and any potential firewall restrictions. Some corporate networks might block API calls, requiring configuration changes or whitelist additions.
Next Steps
After successful implementation:
- Explore more complex queries: Move beyond basic text generation to experiment with advanced features like context awareness and specialized knowledge domains. Try multi-turn conversations and different prompt engineering techniques to optimize results.
- Consider integrating into larger projects: Look for opportunities to incorporate Grok2-beta into existing applications or workflows. This could include chatbots, content generation systems, or automated data analysis tools.
- Join the xAI developer community: Engage with other developers through official forums, Discord channels, or social media groups. Share experiences, learn from others’ implementations, and contribute to the growing knowledge base.
- Stay updated on API changes and improvements: Regularly check xAI’s documentation and release notes for new features or changes. Subscribe to their developer newsletter if available, and follow their social media channels for announcements.
- Plan for long-term usage beyond the promotional period: Develop a strategy for scaling your implementation once the promotional credits end. This includes budgeting for API usage, optimizing query efficiency, and potentially exploring bulk pricing options.
This comprehensive setup guide should help you get started with testing Grok2-beta using either the shell script or Python implementation. Remember to maintain security best practices and regularly monitor your credit usage to make the most of this promotional offer.